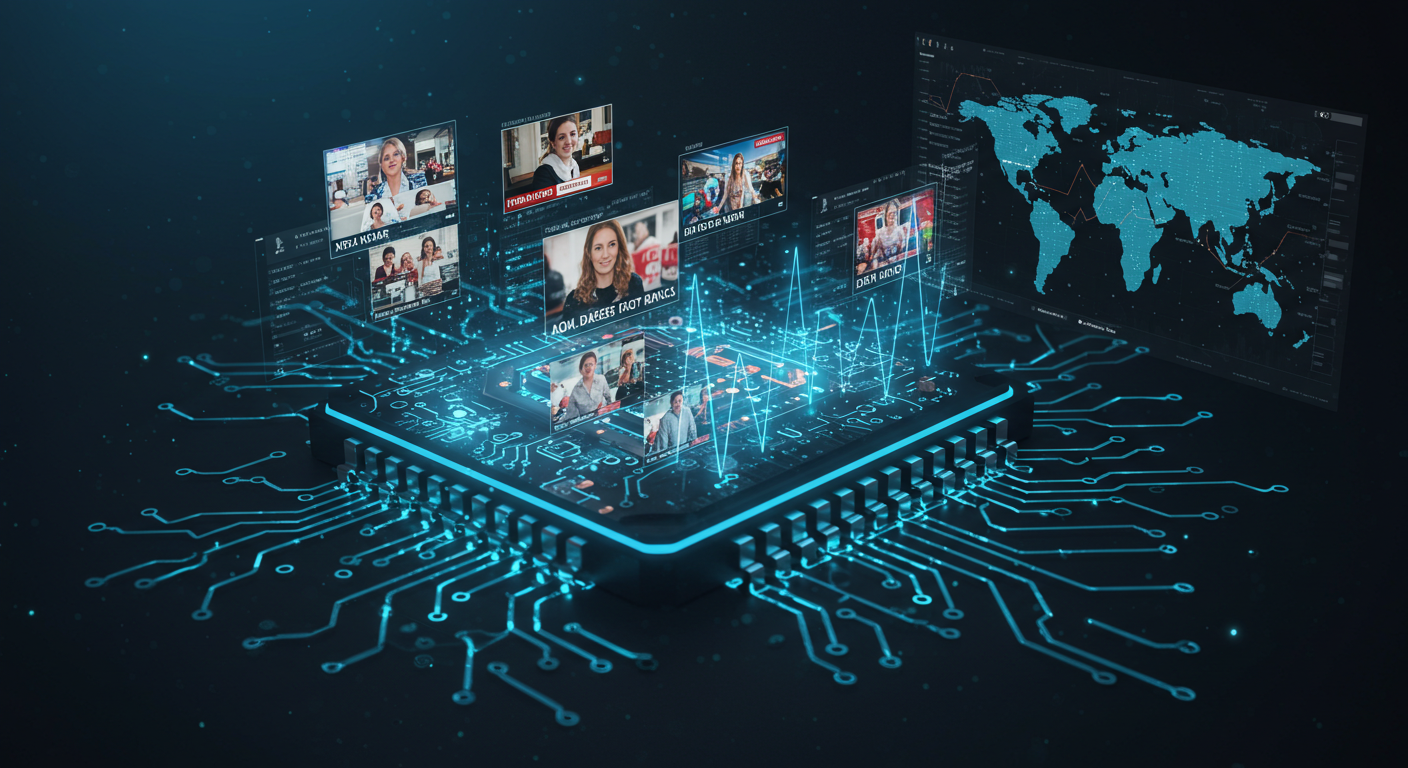
All code on this page is available on GitHub.
Follow us on X for more content like this.
Building Multi-Screen Electron Applications for Enhanced Cognitive Workflows
How modern application architecture can expand your information processing capabilities
Introduction
In today's information-rich environment, our cognitive bandwidth is increasingly overwhelmed. Professionals in finance, healthcare, research, and other data-intensive fields face a common challenge: how to process, visualize, and act upon multiple streams of information simultaneously.
While our brains excel at pattern recognition, they struggle with context-switching and managing multiple data flows. This is where multi-screen applications offer a compelling solution—extending our cognitive capabilities through thoughtful design and spatial arrangement of information.
In this article, I'll walk through how to build a multi-screen Electron application that can help overcome these cognitive limitations by distributing information across physical space, creating an extended mental workspace.
The Power of Distributed Cognition
Before diving into the technical implementation, it's worth understanding why multi-screen setups can enhance cognitive processing:
- Spatial Memory: Our brains are wired to remember information based on spatial location
- Reduced Cognitive Load: Minimizing window switching reduces mental burden
- Parallel Processing: Enabling simultaneous observation of multiple data streams
- Context Preservation: Maintaining visual context for related information
Think of a financial trader watching market movements, news feeds, and portfolio performance simultaneously, or a security analyst monitoring multiple threat vectors across a network—these scenarios benefit tremendously from distributed visual information.
Building Our Multi-Screen Electron Solution
Let's explore how to create a flexible, multi-screen Electron application that can form the foundation for any cognitive augmentation tool. Our example will create a system with six synchronized screens, each capable of displaying different data streams.
Bootstrap Script Overview
I've created a comprehensive bootstrap script that handles the entire setup process. Here's what it accomplishes:
- Creates a new Electron Forge project with webpack support
- Configures React integration for UI components
- Sets up proper webpack configuration for JSX support
- Creates the main process that handles multi-screen detection and window management
- Implements service modules that simulate real-time data streams
- Builds screen components that display and interact with these data streams
The Architecture
The application follows a layered architecture:
- Main Process: Coordinates windows across displays
- Renderer Processes: Screen-specific UI components
- Service Modules: Data processing and business logic
- IPC Bridge: Communication between processes
This separation allows for scalable, maintainable code while supporting complex data visualization needs.
Key Implementation Details
Let's examine the most important parts of our implementation:
1. Multi-Screen Detection and Window Management
The heart of our multi-screen capability lies in the main process:
const { app, BrowserWindow, screen } = require('electron');
const createWindows = () => {
// Get all displays
const displays = screen.getAllDisplays();
const primaryDisplay = screen.getPrimaryDisplay();
// Hardcoded setup for 6 screens (even if fewer are available)
for (let i = 0; i < 6; i++) {
// Use the actual display if available, otherwise use primary
const display = displays[i] || primaryDisplay;
// Create the browser window on the appropriate display
const mainWindow = new BrowserWindow({
x: display.bounds.x,
y: display.bounds.y,
width: display.bounds.width,
height: display.bounds.height,
fullscreen: true,
// ...other configuration
});
// Load the renderer with the screen index as a parameter
mainWindow.loadURL(MAIN_WINDOW_WEBPACK_ENTRY + `?screen=${i}`);
}
};
This code automatically detects all connected displays and creates a full-screen Electron window on each one. If fewer than six physical displays are available, it will create multiple windows on the primary display.
2. IPC Communication
For a multi-screen application to function effectively, the screens need to communicate. We implement this using Electron's IPC (Inter-Process Communication) system with preload scripts:
// In preload.js
const { contextBridge, ipcRenderer } = require('electron');
// Get the screen index from the URL
const urlParams = new URLSearchParams(window.location.search);
const screenIndex = parseInt(urlParams.get('screen')) || 0;
const channel = `screen-${screenIndex}`;
// Expose limited API to renderer
contextBridge.exposeInMainWorld('electron', {
screen: {
index: screenIndex,
},
ipc: {
sendData: (data) => {
ipcRenderer.send(`${channel}:send-data`, data);
},
onReceiveData: (callback) => {
ipcRenderer.on(`${channel}:receive-data`, (_, data) => callback(data));
},
onPushData: (callback) => {
ipcRenderer.on(`${channel}:push-data`, (_, data) => callback(data));
}
}
});
This approach maintains proper security boundaries while allowing screen-specific communication channels.
3. React Components for Each Screen
Each screen renders a unique React component designed for its specific information display:
// In App.jsx
import React, { useState, useEffect } from 'react';
import Screen1 from './Screen1';
import Screen2 from './Screen2';
// ...and so on
const screenComponents = [
Screen1,
Screen2,
Screen3,
Screen4,
Screen5,
Screen6
];
const App = () => {
const [screenIndex, setScreenIndex] = useState(0);
useEffect(() => {
// Get the screen index from the electron global
if (window.electron) {
setScreenIndex(window.electron.screen.index);
}
}, []);
// Render the appropriate screen component based on the index
const ScreenComponent = screenComponents[screenIndex] || screenComponents[0];
return <ScreenComponent />;
};
This pattern allows for specialized components that display information appropriately for each screen's purpose.
4. Service Modules for Data Processing
Each screen is backed by a service module that handles data processing:
// In service1.js
exports.processData = (data) => {
console.log('Service 1 processing data:', data);
// Process the data and return results
return {
source: 'Service1',
receivedData: data,
processed: true,
timestamp: new Date().toISOString()
};
};
// Push real-time data to the screen
exports.startDataPush = (callback) => {
// Push data every 5 seconds
intervalId = setInterval(() => {
const data = {
source: 'Service1',
type: 'push',
value: Math.random() * 100,
timestamp: new Date().toISOString()
};
callback(data);
}, 5000);
};
These modules simulate real-time data processing and streaming, which you would replace with your actual data sources.
Bootstrap Script
The bootstrap script automates the entire setup process, from project initialization to creating all necessary files with proper configurations. Here's how you would use it:
- Save the script as
bootstrap.sh
- Make it executable with
chmod +x bootstrap.sh
- Run it:
./bootstrap.sh
- Navigate to the created directory:
cd multi-screen-electron-app
- Start the application:
npm start
The script creates a functional multi-screen application skeleton that you can adapt for your specific cognitive augmentation needs.
Potential Applications
This multi-screen architecture opens up possibilities for various cognitive tools:
- Financial Analysis: Market data, news feeds, portfolio performance across screens
- Security Operations: Network traffic, threat feeds, system status across physical space
- Research Dashboards: Literature review, data visualization, note-taking in parallel
- Medical Monitoring: Patient vitals, medical history, treatment protocols simultaneously
- Project Management: Task boards, team activities, documentation, and metrics
Conclusion
As we navigate increasingly complex information environments, our cognitive capabilities need technological augmentation. Multi-screen Electron applications offer a practical approach to expanding mental workspace by distributing information across physical space.
The bootstrap script provided here removes the technical barriers to creating such applications, allowing you to focus on the specific cognitive challenges you're trying to solve. By thoughtfully designing the information architecture across screens, you can create powerful tools that extend human cognition beyond its natural limits.
In a world drowning in information, tools that help us process, understand, and act upon that information more effectively aren't just convenient—they're essential cognitive prosthetics for the modern knowledge worker.
Want to explore this further? The complete bootstrap script is available on GitHub. Fork it and adapt it to your specific cognitive augmentation needs.
Security Note: This implementation is intended as a starting point for learning and development purposes. For production applications, you should implement additional security measures including:
- Proper Node.js integration settings (
nodeIntegration: false
) - Context isolation (
contextIsolation: true
) - Content Security Policy (CSP)
- Proper validation of IPC message senders
- Secure window configuration
- HTTPS for loading remote content
- Appropriate permission handling
Always refer to the Electron Security Documentation for best practices before deploying to production.